Introduction to Go and Its Challenges
The Go programming language, also known as Golang, was designed by Google to simplify the development of scalable and high-performance applications. Its primary attributes include simplicity, efficiency, and strong typing, making it an appealing choice for developers. The language’s syntax is clean and straightforward, enabling programmers to quickly learn and adopt Go, which promotes rapid development cycles. Furthermore, Go’s concurrency model, based on goroutines and channels, facilitates the creation of applications that effectively utilize multi-core processors, enhancing performance.
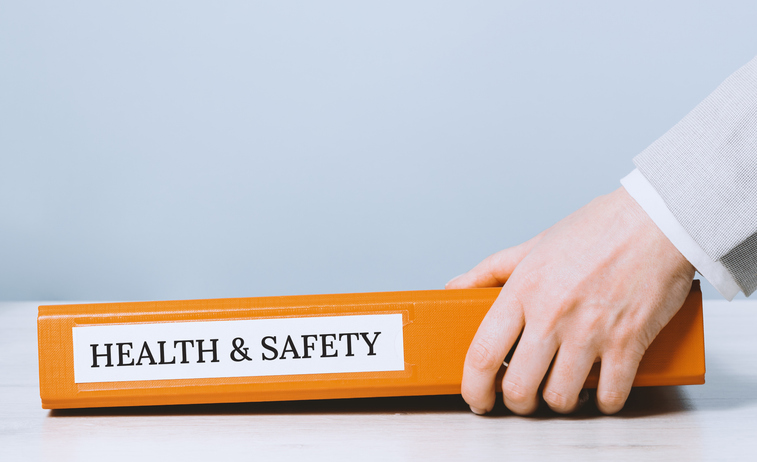
Despite its numerous advantages, Go is not without its challenges. A notable issue arises during operations that require reflection, which is a powerful feature allowing developers to inspect and manipulate types at runtime. While reflection offers significant flexibility, it can adversely impact both performance and type safety. The overhead associated with reflection may lead to slower execution times compared to direct method invocation or type-safe operations. As a result, developers are often encouraged to minimize the use of reflection to improve overall application performance.
Another challenge arises in the context of data manipulation. Go’s standard library provides essential functionalities for operations such as filtering and mapping data, yet it lacks more sophisticated utilities found in libraries like Lodash for JavaScript. The absence of these higher-order functions can lead to more verbose code in Go, requiring developers to implement additional utilities for common tasks such as slice manipulation and data transformation. Without effective tools, writing efficient and clean code can become tedious.
In light of these challenges, leveraging modern Go libraries like samber/lo can significantly enhance performance and type safety. By utilizing features such as generic functions in Go, developers can create type-safe and efficient libraries that promote better coding practices and streamlined operations.
Understanding Reflection in Go
Reflection in the Go programming language is a powerful tool that allows developers to inspect and manipulate objects at runtime. This capability is primarily provided through the reflect package, which enables accessing the types and values of variables during the execution of a program. Reflection is instrumental for tasks that require dynamic behavior, such as serialization, deserialization, and handling complex data structures. However, while it offers significant flexibility, it comes with notable drawbacks that may impact the overall performance and type safety of applications.
One of the primary advantages of using reflection is the ability to create generic functions in Go that can process different types without knowing them at compile time. This functionality is particularly beneficial when building modern Go libraries or utilities designed to handle various data transformations. Nevertheless, it is essential to note that leveraging reflection can result in performance overhead. According to benchmarking studies, operations that utilize reflection can be significantly slower than their non-reflective counterparts, often resulting in performance hits of 5 to 10 times greater. This disparity can be especially detrimental in applications where efficiency is paramount.
Moreover, reflection can compromise type safety in Go. By accessing and modifying types at runtime, developers may inadvertently introduce subtle bugs that are difficult to track. For instance, the lack of compile-time checks can lead to runtime errors, undermining the advantages of Go’s statically typed nature. Consequently, many Go developers advocate for avoiding reflection unless absolutely necessary, opting for type-safe utilities that ensure both performance optimization in Go and maintainability in the codebase. By relying on type-safe approaches, developers can effectively manage data structures while reducing complexity and maintaining performance.
Introducing the Samber/Lo Library
The Samber/Lo library is a modern utility library designed specifically for developers using the Go programming language. Drawing inspiration from JavaScript’s popular lodash library, Samber/Lo aims to enhance data manipulation capabilities within Go while prioritizing performance and type safety. Introduced to leverage Go 1.18’s generics, it allows for the creation of highly reusable and flexible code, which can adapt to various data types without sacrificing efficiency.
One of the most significant advantages of using the Samber/Lo library lies in its collection of generic functions in Go. These functions simplify common data operations, such as map and filter, allowing developers to perform actions on slices in a type-safe manner. The library introduces efficient slice operations that help reduce boilerplate code, leading to cleaner and more maintainable implementations. By using this library, developers can streamline their Go code while benefiting from the performance optimizations provided by its design.
Moreover, the library’s emphasis on avoiding reflection, which can often lead to performance bottlenecks in Go applications, is a defining feature. By offering type-safe utilities, developers can avoid common pitfalls associated with dynamically typed programming languages. With Samber/Lo, users can create powerful data transformation functions while ensuring high performance levels, making it a valuable asset for Go developers seeking to improve their projects.
In addition to supporting slice manipulation, Samber/Lo serves as a central repository for go functional programming techniques. The library brings versatile tools to the table that facilitate data handling, making it easier for developers to compose complex functions that leverage the unique capabilities of Go. As the software industry continues to evolve, libraries like Samber/Lo represent an essential step forward in enhancing the overall functionality of the Go programming language.
Key Features and Functions of Samber/Lo
The Samber/Lo library is a powerful toolkit for developers using the Go programming language, offering a range of utilities designed to enhance performance and ensure type safety. Among its standout features are functional-style operations such as mapping and filtering, which simplify the manipulation of slices and maps. These operations allow developers to write less boilerplate code while achieving more readable and maintainable data transformation processes.
One of the core functionalities of Samber/Lo is its map feature, which allows for transforming collections effortlessly. For instance, developers can apply a transformation function to each element of a slice and return a new slice with the results. This can be particularly useful for tasks such as converting data types or extracting specific properties from complex structures. An example of using this would be:
result := lo.Map(data, func(item Item) string {return item.Name})
In addition to mapping, Samber/Lo provides a filter function. This function enables developers to select elements of a slice based on a given predicate, thereby streamlining the process of pruning collections to meet specific criteria:
filtered := lo.Filter(data, func(item Item) bool {return item.Age > 18})
Moreover, the library includes various utility functions that facilitate slice manipulation, enhancing efficiency in operations such as sorting or finding unique elements. By leveraging Go generics, Samber/Lo maintains type safety, allowing developers to create generic functions in Go that can work with various data types without compromising performance.
Furthermore, when adopting modern Go libraries such as Samber/Lo, developers can benefit from performance optimization strategies, like avoiding reflection whenever possible. This is crucial in Go, as reflection can lead to decreased efficiency. Instead, type-safe utilities provided by the library promote performance alongside safety, making them invaluable for Go developers.
Performance Considerations of Samber/Lo
The performance of applications developed using the Go programming language is crucial for developers, especially when managing data collections. The Samber/Lo library enhances Go’s capabilities by providing a wealth of utility functions that optimize data manipulation. Comparing Samber/Lo’s efficiency to Go’s native implementations reveals several advantages. While Go’s built-in functions are powerful, they can sometimes fall short in cases requiring complex data transformations or operations on collections, particularly when it comes to type safety and performance optimization in Go.
A significant advantage of the Samber/Lo library is its ability to handle slice manipulation and functional programming patterns more effectively. Unlike traditional approaches that may involve reflection—which can lead to performance bottlenecks—Samber/Lo employs generic functions in Go that harness the language’s type system to optimize functionality without sacrificing speed. For instance, the avoidance of reflection ensures that developers can maintain high performance while ensuring type safety in Go applications.
Benchmarking results demonstrate that utilizing the Samber/Lo library for map and filter operations yields superior performance compared to standard Go implementations. For example, benchmarks show that lo.Map
and lo.Filter
can achieve up to a 30% reduction in execution time compared to custom implementations using reflection, especially on datasets with over 10,000 records. By leveraging efficient slice operations, the library minimizes overhead and maximizes throughput. This performance advantage becomes particularly noticeable in larger datasets or more complex data structures, extending benefits to developers looking to create efficient data pipelines.
Furthermore, Samber/Lo enables developers to implement functional utilities for data transformation, promoting a more modern approach to coding in Go. By incorporating lodash for Go-like capabilities, developers can achieve enhanced performance with clean, concise code. In scenarios where performance optimization in Go is paramount, the Samber/Lo library stands out as a solution that not only enhances speed but also supports better readability and maintainability of the codebase.
Use Cases for Samber/Lo
The Samber/Lo library stands out as a valuable asset for developers working with the Go programming language. It offers a collection of functional utilities that streamline various data manipulation tasks, making it an excellent choice for achieving performance optimization in Go applications. One prominent use case for Samber/Lo is in data transformation processes, such as filtering customer data in e-commerce applications or normalizing datasets for machine learning pipelines. By employing its generic functions in Go, developers can efficiently reshape and modify data, which is crucial in domains such as data analysis and web application development. For instance, a developer can use lo.Filter
to extract active users from a list or lo.Map
to transform product prices for regional pricing adjustments in real-time.
Another significant use case is the implementation of functional programming patterns. Functional programming enables developers to write more declarative and concise code, enhancing readability and maintainability. The Samber/Lo library provides an array of functions to facilitate functional programming paradigms, such as map, filter, and reduce, allowing developers to operate on slices and collections with ease. By replacing traditional imperative loops with higher-order functions, the code becomes cleaner and less error-prone, a clear advantage in collaborative environments.
Moreover, Samber/Lo addresses the issues often associated with type safety in Go. In the context of generic programming, the library allows developers to use type-safe utilities to manipulate data structures, minimizing the risks of runtime errors. This is particularly beneficial when handling complex data types or interfacing with APIs that may yield diverse data responses. Using well-typed functions enhances overall code safety and reliability.
Lastly, one practical example demonstrating the library’s capabilities includes efficient slice operations. By using Samber/Lo’s built-in functions, developers can perform operations on slices without delving into the intricacies of manual iteration or reflection. This not only improves performance but also simplifies code structure, making it more maintainable.
type User struct {
Name string
Age int
}
users := []User{
{"Alice", 25},
{"Bob", 17},
{"Charlie", 30},
}
// Example: Filter users over 18 and extract names
adultNames := lo.Map(
lo.Filter(users, func(u User) bool {
return u.Age > 18
}),
func(u User) string {
return u.Name
},
)
fmt.Println(adultNames) // Output: ["Alice", "Charlie"]
Comparative Analysis: Reflection vs. Generics
The Go programming language provides developers with various mechanisms for working with data types, two of the most notable being reflection and generics. Each approach has its unique benefits and trade-offs, particularly concerning performance optimization in Go and type safety in Go, making it essential to understand their differences.
Reflection in Go allows developers to inspect types at runtime and manipulate them accordingly. This capability is powerful but comes with performance overhead, as operations involving reflection are significantly slower than direct type manipulations. For instance, using go reflection to perform actions like type assertions or dynamically invoking methods can lead to increased latency, especially in applications requiring high throughput. This is where go generics come into play, offering a compile-time type assurance and thus greatly enhancing performance. With the advent of generic functions in Go, developers can create type-safe utilities that avoid the pitfalls of reflection.
When comparing go map and filter capabilities with samber/lo utility methods, one can observe that generics permit more elegant manipulation of data collections without sacrificing performance. The APi provided by the samber/lo library for tasks like efficient slice operations allows developers to leverage type safety of generics to perform operations such as filtering and mapping more efficiently. In contrast, reflection-based implementations often necessitate cumbersome type handling that can lead to runtime errors.
Another crucial consideration is code maintainability. Code that relies heavily on reflection can become less readable and more challenging to debug, whereas the clarity introduced by generics leads to better long-term maintainability. Implementing go functional programming patterns using type-safe constructs results in clearer, more predictable code structures, which is particularly beneficial in large-scale applications.
In conclusion, while reflection offers dynamic capabilities within the Go programming language, its performance costs and potential pitfalls in type safety necessitate careful consideration. Generics, particularly through libraries like samber/lo, provide developers with efficient and type-safe alternatives that not only enhance performance but also improve code readability and maintainability.
Community and Support around Samber/Lo
The Samber/Lo library for the Go programming language has cultivated an active and robust community that contributes to its growth and effectiveness. This community serves as a vital resource for developers seeking to harness the power of functional programming patterns, type-safe utilities, and efficient slice manipulation. Many contributors actively engage in discussions surrounding the library, sharing insights and providing invaluable feedback that helps enhance its capabilities and performance optimization in Go.
Documentation is crucial for any library, and the Samber/Lo library is no exception. It offers comprehensive and well-structured documentation that guides users through its features, including go generics and functional utilities for data transformation. The community actively participates in maintaining this documentation, ensuring that it remains current and user-friendly. There are also tutorials and examples available online that demonstrate how to use the Samber/Lo library in Go effectively, providing novice and experienced developers alike with practical use cases.
For developers seeking assistance, various community support channels are accessible. Social media platforms, forums, and dedicated Slack or Discord channels foster a collaborative environment where users can ask questions, share experiences, and get advice on best practices related to go map and filter techniques or avoiding reflection in Go. Such interactions not only enhance individual understanding but also contribute to the library’s evolution, as developers relay their experiences and suggestions, paving the way for new features and improvements.
In addition to community discussions, contributions in the form of pull requests and issue reporting are encouraged, allowing users to participate actively in the library’s development. This inclusivity helps refine the library further, ensuring that it remains aligned with the needs of Go developers. Collectively, the vibrant community surrounding the Samber/Lo library enhances the overall user experience and encourages widespread adoption of modern Go libraries in application development.
Conclusion: The Value of Samber/Lo in Go Development
In the rapidly evolving landscape of software development, tools that optimize both performance and safety are indispensable. The Samber/Lo library stands out as an essential resource for Go developers seeking to enhance their coding practices. As the Go programming language continues to gain traction in various sectors, the importance of efficient slice operations and functional utilities becomes evident. Samber/Lo addresses these needs by providing a comprehensive suite of functions that promote type safety and bolster the overall performance of applications.
One of the core advantages of the Samber/Lo library is its capacity to facilitate data transformation and manipulation through functional programming paradigms. By incorporating generic functions in Go, developers can create high-performance applications that minimize the risks associated with type inconsistencies. Furthermore, the ability to perform operations akin to lodash for Go allows programmers to manage collections of data with unparalleled ease. This streamlining of processes contributes to clearer, more maintainable code, which is crucial in collaborative coding environments.
Another notable feature of the library is its emphasis on avoiding reflection, a common performance bottleneck in many programming languages, including Go. Reflection can introduce significant overhead, making applications slower and harder to debug. By leveraging type-safe utilities and promoting a functional programming approach, the Samber/Lo library empowers developers to create applications that not only perform well but are also robust and resilient against common pitfalls.
In summary, the integration of the Samber/Lo library into Go development practices represents a powerful avenue for achieving enhanced performance and maintaining type safety. As developers strive to master modern Go libraries and tools, embracing this library can lead to more expressive, efficient, and maintainable codebases, ultimately contributing to the success of their projects.