APIs, or Application Programming Interfaces, are the backbone of modern software development. They enable different software applications to communicate and share data, driving the functionality of everything from mobile apps to cloud platforms.
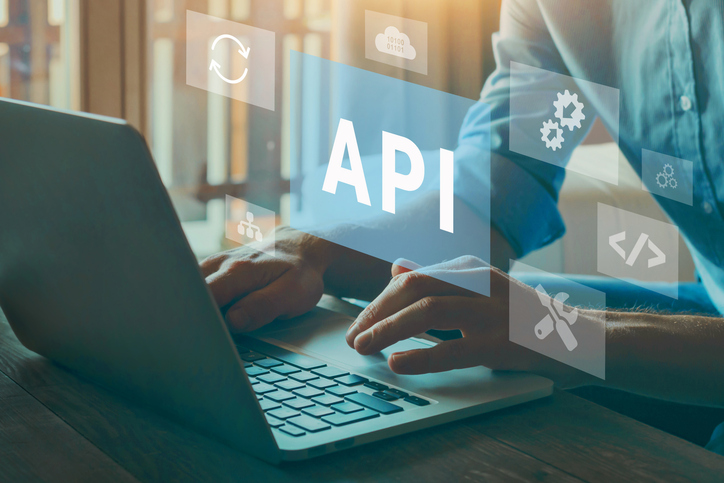
However, implementing APIs is not a straightforward task. It requires a deep understanding of security, performance, and scalability considerations. This is where many tech startup founders and non-tech business owners often struggle.
Security is a critical aspect of API implementation. APIs often handle sensitive data, making them a prime target for cyberattacks. Therefore, it’s crucial to follow secure API design principles, such as data encryption and proper authentication mechanisms.
Performance is another key factor. An API that is slow or unreliable can significantly degrade the user experience, leading to customer dissatisfaction and potential loss of business. Techniques such as efficient error handling and rate limiting can help optimize API performance.
Scalability is equally important. As your user base grows, your APIs need to handle increasing amounts of traffic without compromising performance or security. This is where concepts like microservices APIs and load balancing come into play.
But how can you navigate these complexities if you’re not a tech expert? That’s where this guide comes in. It aims to demystify the technical aspects of API implementation, providing you with actionable insights and best practices.
Whether you’re a tech startup founder trying to make informed decisions for your business, or a non-tech business owner looking to leverage technology for growth, this guide is for you. It’s designed to be accessible and easy to understand, regardless of your technical background.
In the following sections, we’ll delve into each aspect of API implementation in detail. We’ll cover everything from data encryption in APIs and CORS configuration, to API error handling and Cloudflare API protection.
We’ll also explore topics like API scalability, HTTPS for APIs, API performance optimization, and API authentication. Along the way, we’ll provide practical tips and strategies that you can apply directly to your business.
So, are you ready to dive into the world of API implementation? Let’s get started.
Understanding the Importance of API Security
API security is paramount in today’s digital landscape. APIs often serve as gateways to sensitive data and functionalities within a system. If not secured properly, they can become easy targets for cyberattacks, leading to data breaches and unauthorized access.
Securing APIs involves several key practices. First and foremost is implementing robust authentication mechanisms. Authentication ensures that only authorized users can access the API, protecting sensitive information and system resources. OAuth 2.0 is a popular framework for providing secure authorization flows.
Data encryption is another crucial element of API security. By encrypting data both in transit and at rest, you can prevent unauthorized parties from reading sensitive information. Implementing HTTPS for APIs is an effective way to secure data transfer over the network.
Proper CORS (Cross-Origin Resource Sharing) configuration is also essential. CORS headers allow you to control which domains can interact with your API, minimizing exposure to malicious sites. This is especially important in web applications that interact with APIs across different domains.
Here’s a quick checklist of API security measures you should consider:
- Implement strong authentication and authorization
- Use data encryption to protect sensitive information
- Configure CORS to control access across domains
- Regularly update and patch API components
- Conduct security audits and vulnerability assessments
Adopting these practices will help you mitigate risks, protect your users’ data, and maintain trust. Remember, API security is not just a one-time setup but an ongoing process. Regular assessments and updates are crucial to adapting to new security challenges as they arise. By prioritizing security, you can ensure that your APIs remain robust and reliable in a constantly evolving threat landscape.
Secure API Design Principles
Designing APIs with security in mind is a critical step in the development process. Secure API design not only safeguards data but also ensures system integrity. It’s essential to follow principles that minimize vulnerabilities.
One principle is the implementation of the least privilege access model. This approach limits access to API functions based on necessity, reducing potential attack vectors. Only provide access to necessary data and operations for authenticated users.
Error handling also plays a role in security. Avoid exposing stack traces or internal error codes through your API. Detailed error messages can give attackers clues about your system’s structure, making it easier for them to exploit.
Consider the security of data being exchanged. Implement input validation to ensure that only properly formatted data is processed. This practice blocks malicious inputs that could trigger vulnerabilities like SQL injection.
Here’s a list of additional secure design principles to guide your API development:
- Enforce input validation and data sanitation
- Use secure libraries and frameworks
- Regularly review and update API dependencies
- Avoid hard-coding sensitive information
- Keep APIs simple and minimal
Incorporating these principles from the outset helps fortify your API’s security posture. They address both intentional attacks and unintentional failures, providing comprehensive protection. Remember, secure design is not an afterthought; it is an integral part of the API lifecycle.
Data Encryption in APIs
Data encryption is vital in protecting sensitive information exchanged via APIs. It converts data into a coded format, accessible only by individuals with the correct key. This ensures that even if data is intercepted, it remains unreadable.
API encryption typically involves two aspects: data in transit and data at rest. For data in transit, HTTPS is the industry standard. It encrypts the communication channel between the client and the API server, shielding it from eavesdroppers.
Data at rest pertains to information stored in databases or files. Ensuring encryption at this stage prevents unauthorized access to stored data. Encryption keys must be managed securely, as they are the means to decrypt the data.
It’s important to use up-to-date encryption protocols and algorithms. Deprecated methods can expose data to risks, rendering encryption efforts futile. Regularly review encryption strategies and update to the latest standards to maintain robust security.
API Authentication Mechanisms
Authentication ensures that users accessing your API are genuine. It’s a line of defense against unauthorized entities seeking to exploit your system. Effective API authentication can be achieved through various mechanisms.
Commonly, APIs use tokens for authentication. Tokens are identifiers generated upon successful login, allowing clients to authenticate requests without sending credentials repeatedly. This reduces the risk of credential exposure over insecure connections.
API keys are another method, where a unique key is assigned to each client. This allows the API to recognize and authenticate the client directly. However, API keys must be kept secret, as they can grant full access if compromised.
Certificates offer a robust alternative for authentication. Based on Public Key Infrastructure (PKI), they provide a higher level of security. Certificates prove the identity of both the client and server, minimizing the chance of man-in-the-middle attacks.
Implementing Web Application Firewall (WAF)
Web Application Firewalls (WAFs) add an extra layer of security to your APIs. They monitor traffic for suspicious activity, blocking threats before they reach your application. Implementing a WAF protects against common exploits like SQL injection and cross-site scripting.
WAFs operate at the application layer, analyzing HTTP requests and responses. They can identify patterns that indicate malicious activity, providing real-time protection. Configurable rules allow WAFs to adapt to specific threats your API might face.
Deploying a WAF is relatively straightforward, with many cloud-based solutions available. These services offer hassle-free implementation, letting you integrate security without extensive infrastructure changes. They often provide dashboards for monitoring traffic and incidents.
By filtering traffic through a WAF, you can protect both your API and its underlying systems. This helps reduce the burden on developers to manage security manually. WAFs ensure scalability as your API grows, maintaining protection against evolving threats.
OAuth 2.0 and Token-Based Security
OAuth 2.0 is a popular framework that enhances API security through token-based authentication. It enables secure delegated access, allowing third-party applications to interact with your API on behalf of users. This eliminates the need to share sensitive credentials directly.
OAuth 2.0 employs access tokens granted via authorization endpoints. Users authenticate with the service provider, which issues a token for API access. This token has limited scope and lifetime, reducing risks associated with long-term credential storage.
Refresh tokens complement the system, allowing tokens to be renewed without re-authentication. This balance maintains security while enhancing user experience. Token revocation mechanisms further increase security, enabling token invalidation if a breach occurs.
The framework’s flexibility accommodates various authentication scenarios. These include single sign-on (SSO) systems and integrations with identity providers. By leveraging OAuth 2.0, developers provide secure, streamlined access to their APIs, improving both security and user experience.
Best Practices for API Performance Optimization
Enhancing API performance is crucial for delivering a seamless user experience. A high-performing API reduces latency and boosts throughput, essential for scaling applications. Applying performance optimization best practices ensures your API meets these demands.
Start by analyzing your API to identify bottlenecks. Profile API calls to find areas where responses are delayed. This can include inefficient database queries or excessive data processing. Optimizing these bottlenecks leads to significant performance improvements.
Consider the impact of network latency. The physical distance between users and servers can influence response times. Implementing content delivery networks (CDNs) caches API responses closer to end-users, reducing delay.
Efficient data serialization is another vital aspect. Choose lightweight formats like JSON or Protocol Buffers to minimize data transfer times. This reduces payload size and speeds up communication between clients and servers.
Here’s a list of best practices to enhance API performance:
- Utilize compression to reduce payload size
- Optimize database queries and indexing
- Implement client-side caching
- Monitor performance metrics regularly
- Streamline API response structure
By adopting these best practices, your APIs will be well-equipped to handle increased loads without compromising on speed or reliability. A well-optimized API not only satisfies existing users but also scales effectively to accommodate growth.
Efficient API Error Handling
API error handling is an integral component of user experience. A well-crafted error handling strategy provides clear feedback and guides users towards corrective actions. Efficient error handling entails consistency and clarity.
Utilize standardized HTTP status codes to indicate error types. Codes like 404 for ‘Not Found’ and 500 for ‘Server Error’ communicate issues effectively. Coupling these with descriptive messages helps users understand the nature of the error.
Avoid exposing internal system details in error responses. Providing too much information can inadvertently aid attackers in exploiting your API. Balance transparency with security by offering only necessary information.
Consistently logging errors is another essential practice. Comprehensive logs assist developers in identifying and resolving issues swiftly. Ensure that logs capture relevant context, making it easier to trace back errors to their source.
A robust error handling mechanism improves both developer experience and end-user satisfaction. By managing errors effectively, you create a resilient API that responds gracefully to unexpected conditions.
API Rate Limiting Techniques
API rate limiting is crucial for maintaining service quality and preventing abuse. It controls the number of requests a client can make within a set timeframe. Rate limiting protects your API from traffic spikes that could degrade performance.
Choose a rate limiting strategy that aligns with your API’s usage patterns. Fixed window and sliding window algorithms are common methods. Fixed window limits requests in defined intervals, while sliding window averages requests over time.
Define limits based on user roles or subscription levels. This allows more lenient limits for premium users, providing additional value while protecting resources. Adjust these limits dynamically based on real-time traffic analysis.
Communicate rate limits clearly through headers. Provide details of the remaining requests and reset time, helping clients manage their usage. This transparency fosters trust and enables users to adjust their behavior accordingly.
Implementing rate limiting ensures that your API remains available and responsive. It prevents resource exhaustion and enhances security, creating a stable environment for both applications and users.
API Caching Strategies
Caching is a powerful technique for optimizing API performance. By storing and reusing previous responses, caching reduces server load and decreases latency. Effective caching strategies can significantly enhance user experience.
Implement client-side and server-side caching techniques. Client-side caching reduces requests by storing data locally. Server-side caching offloads processing from back-end systems by serving stored responses.
Consider cache invalidation policies. Decide when cached content should expire or be updated. Strategies like time-to-live (TTL) and cache purging ensure data accuracy while benefiting from caching.
Employ HTTP cache headers to guide client behavior. Headers such as Cache-Control and ETag help clients manage their cache, ensuring data consistency and efficiency. By configuring these headers, you streamline the caching process.
By carefully designing and implementing caching strategies, your API can handle high traffic volumes gracefully. Caching enables faster responses, conserves resources, and ensures a smooth, reliable experience for users.
Ensuring API Scalability for Growth
API scalability is pivotal for accommodating increasing user demands. As usage grows, your API must maintain performance and reliability. Scalability strategies ensure your system can handle additional load gracefully.
Begin by analyzing current traffic patterns. Understanding user behavior provides insight into potential growth areas. This analysis informs which scaling techniques to prioritize.
Adopt horizontal scaling to distribute load across multiple servers. Adding resources instead of upgrading existing ones helps maintain performance during peak usage periods. This approach provides flexibility and resilience.
Integrate automated scaling tools. Monitor API metrics to adapt resource allocation dynamically. Automation ensures optimal resource use without manual intervention, crucial for efficiently managing varying traffic loads.
Key practices for enhancing API scalability include:
- Implementing caching and load balancing
- Designing modular and decoupled architectures
- Employing containerization for resource management
- Utilizing cloud services for scalable infrastructure
- Regularly reviewing and optimizing code
By embedding these practices into your API strategy, you’ll create a robust system capable of supporting growth. Scalability ensures your API remains responsive and reliable, regardless of demand fluctuations.
Microservices APIs and System Architecture
Designing APIs with a microservices architecture enhances scalability and flexibility. By breaking down applications into smaller, independent services, you optimize both development and operations.
Microservices APIs allow different components to scale independently. If one service experiences increased load, scale just that service rather than the entire application. This modularity leads to efficient resource use.
Emphasize clear communication between services. Use lightweight protocols like RESTful APIs or gRPC for inter-service messaging. This approach ensures smooth interaction while minimizing latency.
Implement service discovery to manage communication dynamically. As new services are added or removed, discoverable endpoints simplify integrations. This adaptability accelerates deployment and enhances scalability.
Microservices architecture promotes agile development and deployment. By focusing on small, focused services, your team can innovate and iterate swiftly. This agility is key to responding to growth and evolving user needs.
Load Balancing and API Gateways
Load balancing is vital for distributing client requests evenly. This process ensures no single server becomes overwhelmed, maintaining consistent performance.
Different load balancing algorithms offer varying benefits. Round-robin distributes requests sequentially, while least connections routes to the server with the fewest active connections. Choose the strategy that aligns with your system’s needs.
API gateways serve as a single entry point for client requests. They manage traffic, enforce security policies, and streamline communication. Gateways reduce complexity and improve scalability by centralizing API management.
Consider using API gateways for protocol translation. Transform requests and responses to suit different client needs seamlessly. This flexibility supports diverse clients and devices without additional development effort.
Effective use of load balancing and API gateways allows seamless scaling. These components adapt to traffic changes, protecting against service outages and ensuring user satisfaction.
Cloud-Based Solutions and Cloudflare API Protection
Cloud-based solutions offer scalable infrastructure as a service. Leveraging the cloud allows you to scale operations in response to demand effortlessly. Resources can be allocated dynamically, optimizing costs and performance.
Using cloud platforms, deploy geographically distributed data centers. This setup minimizes latency and ensures high availability. Users experience fast, reliable connections, regardless of their location.
Cloudflare offers robust API protection tools. Its services shield APIs from DDoS attacks and other threats. By deploying Cloudflare protection, you safeguard your API while maintaining performance.
Integrate advanced security features, such as automated threat detection and mitigation. Cloudflare’s API protection adapts to changing threats, minimizing potential damage. This real-time defense is crucial for maintaining user trust.
Cloud-based solutions paired with Cloudflare protection create a resilient API ecosystem. This combination supports growth and security, delivering a dependable service to your users.
API Documentation and Developer Experience
API documentation significantly impacts the developer experience. Clear and comprehensive documentation enables seamless integration with your API, fostering adoption.
Organize your documentation to guide developers through the onboarding process. Detail each endpoint’s purpose, expected input, and response format. This clarity reduces integration time and errors.
Use interactive documentation tools to enhance understanding. Tools like Swagger UI allow developers to test endpoints directly within the documentation. This interactivity improves developer engagement and efficiency.
Maintain up-to-date documentation. As your API evolves, ensure your documentation reflects these changes. Keeping information current avoids confusion and supports ongoing developer success.
To enhance developer experience through documentation, consider:
- Using visual aids and code examples
- Providing step-by-step integration guides
- Offering a comprehensive FAQ section
- Facilitating community feedback mechanisms
- Keeping an API changelog for transparency
By focusing on excellent documentation, you empower developers to use your API effectively, driving both adoption and satisfaction.
Leveraging OpenAPI/Swagger Documentation
OpenAPI/Swagger documentation provides a standardized approach to API documentation. This framework enhances consistency and comprehensibility, key for reducing integration complexity.
Begin by generating an OpenAPI specification document. This structured file acts as a single source of truth for your API. Include detailed information about each operation, parameter, and data model.
Swagger UI then transforms your specification into an interactive experience. Developers can explore your API visually and test endpoints in real time. This interaction bridges the gap between documentation and practical use.
Ensure your Swagger documentation is well-organized. Group related endpoints logically, making it easy for developers to locate necessary information. Consistent organization fosters intuitive navigation and understanding.
Regularly update your OpenAPI specification as your API evolves. This practice ensures that your documentation remains accurate and a dependable resource for all developers.
API Versioning Best Practices
API versioning is crucial for managing changes and maintaining compatibility. As your API evolves, versioning ensures existing integrations remain functional.
Establish a clear versioning strategy early on. Decide whether to adopt semantic versioning or a custom approach. Consistency in versioning helps developers anticipate and adapt to changes.
Communicate versioning changes transparently. Notify users of deprecated features and upcoming modifications. This transparency builds trust and allows developers to plan adjustments.
Use URI paths or request headers to indicate API versions. Each method has pros and cons, so choose based on your system’s architecture and developer preferences. Ensuring versions are distinguishable helps in managing calls efficiently.
Regularly review your versioning policy. As your API grows, adapt your strategy to meet new challenges. An agile approach to versioning supports long-term scalability and compatibility.
By implementing these versioning practices, you maintain a stable API ecosystem. This reliability fosters developer confidence and promotes continued use of your API.
Monitoring, Analytics, and Continuous Improvement
Monitoring and analytics are critical in maintaining an API’s performance and security. They allow you to gather insights and identify issues promptly.
To achieve effective monitoring, deploy robust tools that track API usage and performance metrics. These tools should give you a holistic view of how your API is functioning.
Analytics help in understanding usage patterns. By analyzing data, you can identify popular endpoints, peak usage times, and potential bottlenecks. This knowledge aids in optimizing your resources and planning for capacity increases.
Continuous improvement involves iterating based on insights gained from analytics. Update and enhance your API regularly to address inefficiencies and new user requirements.
To support your monitoring and analytics efforts, consider these tools and practices:
- Real-time monitoring platforms like Grafana or Prometheus
- Implementing logging for detailed transaction records
- Using synthetic monitoring for testing endpoints
- Conducting root cause analysis for recurring issues
- Incorporating user feedback into your improvement process
By integrating these elements, you create a proactive environment for API management. This approach ensures your API remains resilient and relevant.
API Analytics and Monitoring Tools
API analytics and monitoring tools provide invaluable insights for maintenance and improvement. They enable you to collect real-time data and make informed decisions.
Start by selecting a tool that fits your needs. Consider options like Google Analytics, Datadog, or Amazon CloudWatch. These platforms offer diverse features and integrations.
Once selected, configure your monitoring tool to track important metrics. Monitor response times, error rates, and throughput. This data uncovers performance trends and potential issues.
Alert systems are another vital feature. Set up alerts for predefined conditions, like slow response times or high error rates. Prompt alerts facilitate swift intervention and resolution.
Regularly review data collected by your monitoring tools. Use these insights to refine your API, enhancing both performance and user satisfaction. This cyclical process supports continuous improvement.
The Role of API Testing in Security and Performance
API testing is foundational in ensuring robust security and optimal performance. It identifies vulnerabilities and performance hiccups before they affect users.
Begin with functional testing to ensure each endpoint behaves as expected. This verification guarantees that your API delivers correct and consistent outputs.
Security testing follows, focusing on identifying vulnerabilities and potential exploits. Check for OWASP vulnerabilities and ensure all security measures, such as authentication, are intact.
Performance testing is crucial for gauging your API’s efficiency. Conduct load tests to assess how your API handles varying traffic levels. This insight helps in optimizing resource allocation.
Combine these testing methodologies for comprehensive coverage. Integration testing can also verify that your API works well with other systems. Thorough testing ensures your API meets quality standards and user expectations.
By embedding testing throughout your development process, you bolster your API’s reliability and effectiveness. Consistent testing routines lead to continual API enhancement and user satisfaction.
Conclusion: Integrating Best Practices into Your API Strategy
Incorporating best practices into your API strategy is essential for sustained growth and reliability. With a focus on security, performance, and scalability, your API can become a robust tool.
Begin with securing your APIs to protect data and ensure compliance. Implement encryption, authentication, and monitoring for a comprehensive security framework.
Performance optimization is equally important. Use rate limiting, caching strategies, and error handling to enhance user experience and maintain service quality.
Scalability enables your API to manage increased demand smoothly. Consider microservices, load balancing, and cloud solutions for flexible and efficient scaling. By consistently integrating these best practices, your API strategy will support your business objectives and adapt to evolving needs.
Final Checklist of API Implementation Best Practices
A final checklist ensures you have covered all vital aspects of API implementation. Use this as a guide to strengthen your API strategy:
- Secure data with encryption and authentication methods.
- Optimize performance through caching and error handling practices.
- Ensure scalability using microservices and load balancing techniques.
- Document your API using OpenAPI or Swagger for clarity and ease of use.
- Implement monitoring and analytics to gather insights and drive improvements.
Regularly reviewing this checklist helps in maintaining a high standard for your API. This approach ensures your systems remain secure, efficient, and scalable.
Encouraging a Culture of Security and Performance
Fostering a culture focused on security and performance is key to a successful API strategy. This mindset should permeate your team and organizational practices.
Start by training your team on the importance of these principles. Provide ongoing education to keep everyone informed of the latest threats and techniques.
Integrate security and performance goals into your development processes. Encourage collaborative efforts between teams to identify and resolve issues effectively.
Celebrate successes and learn from failures. Share insights and improvements with the entire organization to reinforce these values.
By nurturing a culture that prioritizes security and performance, you lay the foundation for a resilient and agile API ecosystem that meets both business objectives and user expectations.