At the heart of OOP are several foundational principles that distinguish it from other programming paradigms, such as structured or functional programming. Understanding these concepts is essential for anyone looking to leverage OOP effectively.
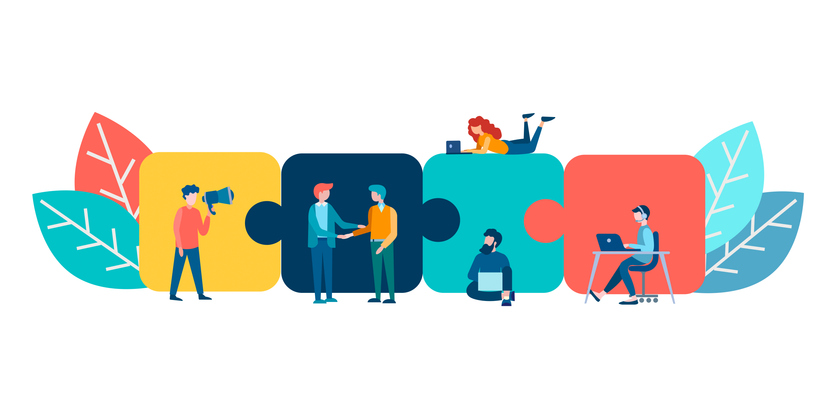
Classes and Objects
Classes and objects are the building blocks of OOP. A class serves as a blueprint for creating objects, which are instances of classes. This blueprint defines the properties (attributes) and behaviors (methods) that the objects created from it will have.
For example, consider a class Car with attributes such as color and make, and methods like drive() and brake(). An object created from this class, say myCar, would inherit these attributes and methods, allowing for specific instances of Car to be created and manipulated. This is akin to having a mold that can create identical shapes but with unique specifics, like color.
Classes promote organization by grouping related functionalities together. This grouping allows developers to logically structure their code, making it easier to locate and modify when necessary. Objects, on the other hand, make the software dynamic, as they can be created, manipulated, and destroyed as needed.
Inheritance
Inheritance allows a new class to adopt the properties and methods of an existing class. This promotes code reusability and establishes a relationship between parent and child classes. For instance, a class ElectricCar can inherit from the Car class, inheriting its attributes and methods while introducing new features specific to electric vehicles.
Inheritance not only helps in code reusability but also in creating a hierarchical structure for classes. This hierarchy allows for more organized code management and understanding, especially in large systems. For example, in a company, you might have a general Employee class and more specific classes like Manager and Developer that inherit from Employee.
Moreover, through inheritance, you can override methods to provide specific implementations in the child class. This allows flexibility and customization, ensuring that each class can behave uniquely while still sharing a common structure. The concept of overriding also aids in maintaining the code base since changes in the parent class can automatically reflect in the child classes.
Encapsulation
Encapsulation involves bundling the data (attributes) and the methods that operate on the data into a single unit or class. It restricts direct access to some of an object’s components, which can prevent the accidental modification of data. This principle is crucial for maintaining the integrity of the object’s data and is implemented through access modifiers like private, protected, and public.
Encapsulation acts as a protective shield that prevents the code from being accessed by unintended parties. This is critical in protecting the internal state of an object from unintended interference and misuse, thereby reducing bugs and enhancing security. For instance, a banking application might encapsulate account details to prevent unauthorized access.
Access control through encapsulation also allows for the implementation of validation logic within methods. This ensures that any changes to an object’s state meet certain criteria before being applied, which can prevent errors and maintain consistency. Encapsulation thus encourages a more disciplined approach to coding, promoting cleaner and more reliable code.
Abstraction
Abstraction focuses on hiding complex implementation details and showing only the essential features of an object. It simplifies the interaction with complex systems by providing a clear interface. For example, when you drive a car, you don’t need to understand the engine’s mechanics; you only need to know how to use the accelerator, brake, and steering wheel.
Through abstraction, developers can reduce programming complexity and effort. By focusing on what an object does rather than how it does it, abstraction helps in managing complexity by breaking down the system into smaller, more manageable units. This is particularly beneficial in large systems where managing every detail would be overwhelming.
Moreover, abstraction allows for the development of more generic and reusable code components. By providing a standard interface, different parts of a system can interact without needing to know each other’s internals, fostering a modular architecture. This not only enhances code reusability but also facilitates easier updates and maintenance.
Polymorphism
Polymorphism enables objects to be treated as instances of their parent class. It provides a way to perform a single action in different forms. A common example is method overriding, where a child class provides a specific implementation of a method already defined in its parent class.
Polymorphism enhances flexibility and integration in programming. It allows for designing systems where classes can interact seamlessly, even if they are from different hierarchies. For instance, in a graphics program, a single function can be used to draw different shapes like circles and squares by leveraging polymorphism.
In addition, polymorphism supports dynamic method dispatch, which means that the method that gets executed is determined at runtime. This capability allows for more dynamic and adaptable code, as the exact method to invoke can be decided based on the object type. Polymorphism thus plays a critical role in achieving loose coupling in code, promoting better design practices.
Advantages and Disadvantages of OOP
OOP offers numerous benefits that can significantly enhance software development practices.
Advantages
- Modularity and Reusability: The use of classes allows for modular code that can be reused across different programs, reducing redundancy and enhancing productivity. Modularity breaks down complex systems into manageable units, promoting easier understanding and maintenance.
- Maintainability: By using encapsulation, OOP makes it easier to maintain and modify existing code without affecting other parts of the program. This is crucial for evolving systems, where changes are inevitable and can be managed without disrupting the whole system.
- Scalability: OOP facilitates the creation of scalable systems due to its structured approach to building software components. This structured approach allows systems to grow and evolve more naturally, accommodating new requirements without extensive rewrites.
- Real-World Modeling: OOP’s focus on objects makes it easier to model real-world scenarios in software, leading to more intuitive and effective designs. This alignment with real-world paradigms simplifies the design process and results in more relatable and understandable software solutions.
Disadvantages
Despite its advantages, OOP is not without its drawbacks:
- Complexity: OOP can introduce additional complexity, especially for simple programs or developers new to the paradigm. This complexity arises from the need to understand and properly implement OOP principles, which can be daunting without proper guidance and experience.
- Performance Overhead: The abstraction and encapsulation that OOP offers can sometimes lead to performance overhead compared to procedural programming. This overhead can be a concern in performance-critical applications, where the additional layers introduced by OOP might impact efficiency.
- Learning Curve: For developers accustomed to procedural or functional programming, transitioning to OOP requires a significant shift in mindset. Understanding concepts like inheritance and polymorphism can be challenging initially, requiring time and practice to master.
OOP Languages and Real-World Examples
Several programming languages support OOP, each with its own syntax and features. Notable examples include Java, Python, C++, and Ruby.
OOP in Java
Java is renowned for its robust OOP features. It supports all core OOP principles and is widely used in enterprise environments for building scalable applications. Java’s strong type system and garbage collection contribute to building reliable and efficient software.
Java’s platform independence, due to the Java Virtual Machine (JVM), makes it a popular choice for cross-platform development. This allows developers to write code once and run it anywhere, enhancing productivity and reach. Moreover, Java’s extensive libraries and frameworks provide a rich ecosystem for developing a wide range of applications.
Java also emphasizes security and performance, making it a preferred choice for large-scale enterprise applications. Its robust security features help in building secure applications, while its performance optimizations cater to the needs of demanding environments.
OOP in Python
Python’s simplicity and readability make it an excellent choice for those new to OOP. It supports all major OOP concepts and is often used in web development, data analysis, and artificial intelligence. Python’s dynamic typing and concise syntax encourage rapid development and prototyping.
Python’s extensive standard library and third-party modules make it a versatile language for various applications. Its popularity in data science and machine learning is driven by libraries like NumPy, Pandas, and TensorFlow, which simplify complex tasks. Furthermore, Python’s community support and resources make it accessible for beginners and experts alike.
Python’s flexibility and ease of integration with other languages also contribute to its widespread use in diverse domains. Its ability to act as a glue language allows for seamless integration with systems written in different languages, enhancing its utility in mixed environments.
OOP in C++
C++ is a powerful language that combines the features of both procedural and object-oriented programming. It is widely used in system/software, game development, and real-time simulations. C++ offers fine-grained control over system resources, making it ideal for performance-critical applications.
C++’s support for low-level programming features, such as pointers and memory management, provides developers with the tools needed to optimize performance. This makes C++ a preferred choice for developing operating systems, drivers, and embedded systems. Additionally, C++’s Standard Template Library (STL) provides a rich set of template classes and algorithms for efficient coding.
Despite its complexity, C++’s flexibility and performance capabilities make it a valuable language for high-performance applications. Its ability to combine procedural and object-oriented paradigms offers developers a wide range of programming techniques to solve complex problems.
Practical OOP Examples
Let’s look at a practical OOP scenario to illustrate its benefits. Consider a software system for a library.
Classes and Objects in Action
- Class: BookAttributes: title, author, isbnMethods: checkout(), returnBook()
In this system, the Book class encapsulates the attributes and methods related to a book. This allows for managing book-related data and operations in a structured manner. For example, the checkout() method can update the book’s status and manage borrowing records.
- Class: MemberAttributes: name, membershipIdMethods: borrowBook(), payFine()
The Member class handles all member-related functionalities. By encapsulating member data and operations, the system can efficiently manage membership activities. This separation of concerns ensures that changes in one class do not affect the other, promoting maintainability.
The use of OOP makes it easier to add new features, such as a DigitalBook class, without disrupting existing functionality. This extensibility is a key advantage of OOP, allowing for the seamless introduction of new components and features as the system evolves.
Interaction Between Classes
Interactions between different classes illustrate the power of OOP in creating dynamic systems. For instance, when a Member borrows a Book, methods from both classes interact to update records and manage transactions. This interaction is facilitated by defining clear interfaces and relationships between classes.
Furthermore, the introduction of new classes, like an eBook class, can be done with minimal impact on the existing system. By inheriting from the Book class, eBook can extend functionalities while maintaining compatibility with existing workflows. This flexibility demonstrates OOP’s capability to adapt to changing requirements and technologies.
Real-World Extensions
In real-world applications, OOP’s principles can be extended to more complex systems. For example, a library system might include classes for managing library staff, inventory, and events. Each class handles specific functionalities, promoting a modular and organized codebase.
Moreover, by leveraging polymorphism, the system can handle different types of Books uniformly, whether they are physical or digital. This uniformity simplifies the code and enhances its readability, making it easier for developers to understand and modify.
OOP vs. Structured Programming
OOP and structured programming differ significantly in their approach. While structured programming focuses on functions and procedures, OOP centers around objects and data. This shift in focus allows for more modular, reusable, and maintainable code but may add complexity that isn’t necessary for all projects.
Function-Centric vs. Object-Centric
Structured programming revolves around functions and procedures, emphasizing a linear flow of control. It is ideal for small, simple programs where a clear sequence of operations is needed. However, as programs grow in size and complexity, this approach can lead to tangled code and difficulties in maintenance.
In contrast, OOP focuses on objects that encapsulate data and behavior, allowing for more flexible and scalable designs. By organizing code around objects rather than functions, OOP promotes a more intuitive and manageable structure, especially in large applications.
Code Reusability and Modularity
OOP’s emphasis on code reusability and modularity is a key differentiator from structured programming. While structured programming can achieve reusability through functions, OOP takes it further by enabling the reuse of entire classes and hierarchies. This reduces redundancy and enhances productivity, particularly in complex systems.
Moreover, OOP’s modularity allows developers to break down large applications into smaller, interconnected components. This modular approach facilitates easier debugging, testing, and maintenance, improving the overall quality and reliability of the software.
Evolution and Adaptability
OOP’s ability to evolve and adapt to changing requirements is a significant advantage over structured programming. In structured programming, adding new features or modifying existing ones can be challenging, often requiring extensive changes to the codebase.
With OOP, new features can be introduced through inheritance and polymorphism without disrupting existing functionality. This adaptability makes OOP ideal for modern software development, where systems must continuously evolve to meet new challenges and opportunities.
Conclusion: The Path from WTF to OMG
Object-Oriented Programming may seem daunting at first, but understanding its principles can revolutionize your software development approach. By utilizing classes, objects, and the core tenets of OOP, you can design systems that are both efficient and scalable.
For developers, mastering OOP can lead to more organized, reusable, and maintainable code, enhancing productivity and reducing errors. For businesses, embracing OOP can result in more robust and adaptable software solutions, better aligning with strategic goals and customer needs.
Whether you’re a tech startup founder navigating the complexities of software development or a non-tech business owner seeking to leverage technology, mastering OOP can be a game-changer in your digital journey. Its principles not only simplify programming but also open new opportunities for innovation and growth, transforming confusion into clarity and empowerment.